Weaving Geography
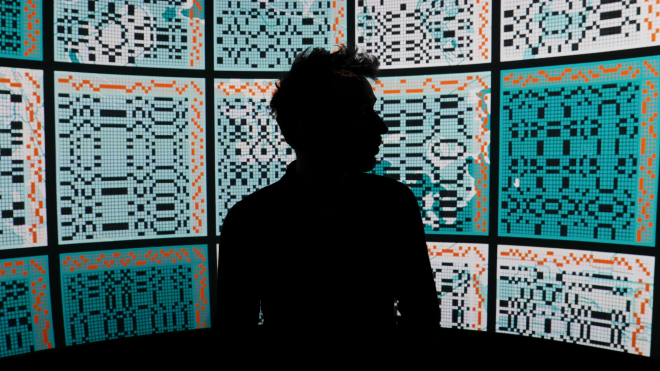
The genesis of this project comes from my earlier research in nuclear semiotics, the original idea was to find a resilient medium to store geographical coordinates and pass them on through cultural craft rather than cold archiving. The chosen locations to encode would be nuclear waste facilities, nuclear accident sites, and other environments within which radioactive waste is stored and will be decaying over the next million years. By that time, the original textile will have disintegrated, but the practice of its weaving would have been passed down generations, carrying down the line copies of the same information; warning further generations about the existence and location of radioactive sites.
The practice of embedding geographic coordinates in cultural artifacts is not new, it is ingrained in human history. From slave songs that discreetly conveyed directions to safer places, to people today purchasing custom laser-cut necklaces that abstract the location where a significant life event took place, encoding places into art and objects is a recurring theme across cultures. I thought it could be interesting to see what applications of this could be used to rethink contemporary geography.
Textile Programming Language
Textile weaving is often considered a precursor to programming. The automation of its labour has evolved through increasingly complex machines, such as the Jacquard loom, which used punch cards as inputs, similarly to early computers. In essence, weaving is the story of two threads and how they intertwine : First the warp, the long vertical thread in which the tension is held, it is the foundation of the fabric. Secondly, the weft, horizontal threads that are woven over and under the warp threads, together they interlace to form the fabric.
Between the 19th and 20th century, the language of weaving has slowly standardised using non-verbal instructions, allowing weavers from across the world to easily communicate patterns to one another. These can take the shapes of 2-dimensional grids, simply indicating when weft threads should go over or under the warp.
Weaving Pattern Instructions
The actual instructions are a little more complicated than the above, as they also give information on how the loom (weaving device) should be configured and used. To write a pattern, there are two set of instructions that we need to provide: the threading and the treadling.
The threading is represented by a horizontal grid, which shows how each warp thread is attached to a shaft (wooden frame). Each line on the grid corresponds to a shaft, and the coloured squares on the line indicate which warp thread is attached to that shaft. The process of connecting the threads to the treadle is called tie-up. Next, the treadling refers to the order in which the shafts are lifted as the weft thread passes through. Since the shafts are controlled by pedals, the treadling is essentially a pedalling sequence.
In the example below, we see weaving instructions for a two-shaft loom controlled by two pedals. Each shaft's threading is represented by two lines, and the pedalling order is shown in two columns. The warp threads alternate between being attached to the first and second shafts, while the pedalling instructions also alternate between both pedals, creating a chequered pattern (note that some shafts and pedals can remain unused if necessary).
The 2x2 orange grid on the top right is called the tie-up, and determines which pedal is connected to which shaft. For simplicity I applied a straight tie-up, meaning that the first pedal raises the first shaft and the second pedal raises the second shaft.
Geo-Coordinate Patterns
Geographical locations can be communicated in many different ways. For instance, saying "through the garden, behind the cherry tree, and around the stone wall" is a valid direction, as long as both of us share the knowledge of my grandmother’s garden. However, when sharing geographic locations on a large scale, the scientific standard is the Latitude-Longitude system, as it references entire earth sphere by drawing horizontal and vertical lines along its surface, and identifying the points where these lines meet.
A standard pair coordinates such as [61.235148, 21.481852], can represent a unique position. In our case, a location next to the city of Olkiluoto, in Finland, pinpointing the Onkalo project, the Finnish nuclear waste repository. In the following section, we will use these coordinates as an example to demonstrate how to convert them into the following pattern:
The map above is interactive, you can zoom, unzoom and click anywhere to display the corresponding weaving pattern calculated using the lattitude and longitude of the selected point. For each point there exists a unique associated pattern. The code is open-source and downloadable here.
Technical Implementation
Algorithm Breakdown
Normalising the coordinates
Converting to whole numbers
Converting to base 4
Applying a palindrome effect
Pseudo code
// Step 1: Normalizing Coordinates
let latitude = 61.235148;
let longitude = 21.481852;
latitude = map(latitude, -90, 90, 0, 180); // Output: 151.235148
longitude = map(longitude, -180, 180, 0, 360); // Output: 201.481852
// Step 2: Converting to Whole Numbers
latitude = latitude * Math.pow(10, 7); // Output: 1512351480
longitude = longitude * Math.pow(10, 7); // Output: 2014818520
// Step 3: Base 4 Conversion
function convertToBase4(decimalNumber) {
let base4 = '';
while (decimalNumber > 0) {
let remainder = decimalNumber % 4;
base4 = remainder + base4;
decimalNumber = Math.floor(decimalNumber / 4);
}
return base4;
}
let latitudeBase4 = convertToBase4(latitude); // Output: 1122021022123320
let longitudeBase4 = convertToBase4(longitude); // Output: 1320011323003120
// Step 4: Creating a Palindrome Pattern
function createPalindrome(sequence) {
let mirroredSequence = sequence.split('').reverse().join('');
return sequence + mirroredSequence;
}
let latitudePattern = createPalindrome(latitudeBase4);
// Output: 11220210221233200233212201202211
let longitudePattern = createPalindrome(longitudeBase4);
// Output: 13200113230031200213003231100231
Explanations
Step 1 : Normalising the coordinates
Latitude ranges from -90 to 90, and longitude ranges from -180 to 180. To work only positive values, the coordinates need to be remapped. The latitude is remapped from -90 to 90 to a range of 0 to 180, and the longitude is remapped from -180 to 180 to a range of 0 to 360. This remapping can be done using the Javascript map()
function.
Step 2 : Converting to whole numbers
To avoid floating-point numbers, the coordinates are multiplied by 10^7 converting them into integers.
Step 3 : Converting to base 4
The coordinates are then converted from base-10 to base-4 to simplify the weaving process, which is based on a 4-shaft loom. The base-4 conversion is achieved by repeatedly dividing the number by 4 and recording the remainders, which form the base 4 number as described in the convertToBase4()
function defined in the pseudo code.
Step 4 : Applying a palindrome effect
To ensure the coordinates can be read from any side of the fabric, I doubled the coordinates and mirrored the second set. Similarly to how the word "KAYAK" reads the same forward and backward, the coordinates will be readable using any fabric orientation.
Mapping the new Coordinates to Weaving Instructions
To preserve a sense of two-dimensionality between the textile and the earth's surface, I decided to map the longitude on the threading grid and the latitude on the treadling grid. Taking the latitude in base 4 palindrome format (11220210221233200233212201202211), we sequentially attach each warp thread to the corresponding shaft. For example, since the number starts with "1", we attach the first warp thread to shaft number 1 (which is the 2nd shaft when counting from zero).
For the pedalling instructions, we take the longitude in base 4 palindrome format (13200113230031200213003231100231). The treadling will follow this sequence from top to bottom, where each number represents the pedal to press. The first number is "1" again, corresponding to pedal number 1 (the 2nd pedal when counting from zero). Following these instructions will give us the final weaving pattern:
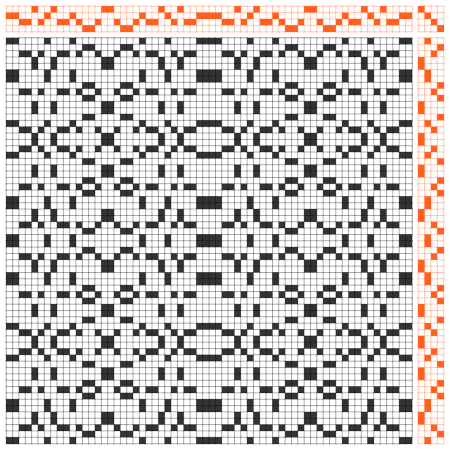
Real-Life Application
To test-case the technical implementation I used the coordinates of France's deep geological nuclear waste repository, the Cigéo project initiated by ANDRA (the french national radioactive waste management agency) in the commune of Bure, Meuse. For this part of the project I collaborated with Camille Ferrer, a weaver and textile designer who studied at l'ENSCI.
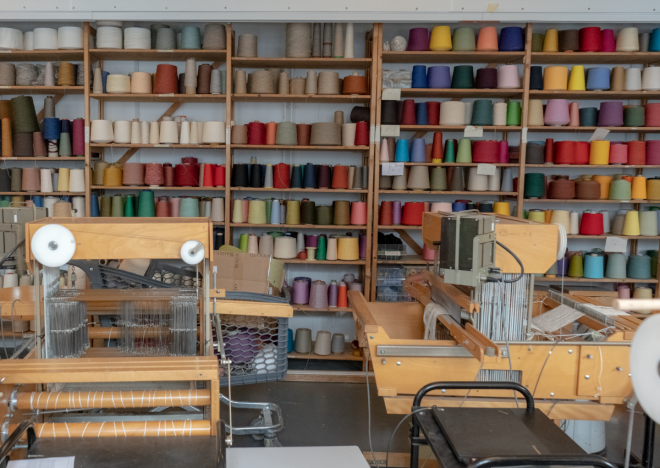
The longer part of the work was translating the knowledge from expected computer behaviour to actual physical textile weaving machines. We realised it was inevitable that we would need to adjust the design if we wanted it readable by the naked eye. The algorithmically generated pattern had significant weft floating (threads skipping several warp threads), with some floats as long as 8 cm. To fix the fragility of the textile, we added thin grey threads of plain weave between each horizontal pattern. This reinforced the structure without altering the underlying mathematical concept.
As for materials, we wanted to choose something that stood out and referenced nuclear waste. Initially, we considered using glass fiber, referencing the vitrification process used for nuclear waste, or basalt stone fibre, referencing the geological storage of the waste. In the end we chose triple-stranded copper wires, as a hint to the electrical infrastructure and the way power lines are transmitted in three-phase systems.
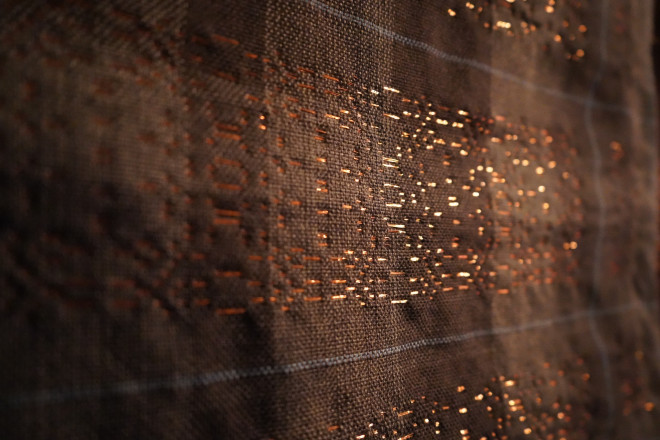
On the other hand this meant that our pattern drawn by copper would have two very distinct sides, on the picture above you can see the front side, while on the bottom you can see the back side with all the floating copper threads.
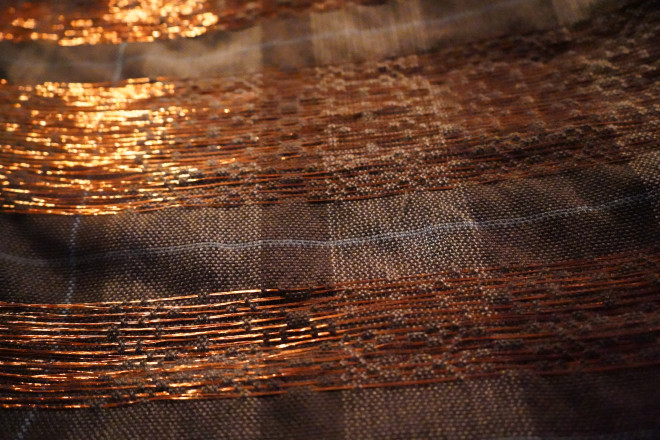
The final piece was exhibited along with the interactive code seen above and the technical implementation. Unfortunately due to the bendy nature of copper, I can not wear the project as a scarf in my everyday life, but I would gladly start over with more standard threads. If you represent a nuclear waste agency (or a textile design school), and would be interested in funding the project further, I would love to develop some new research axis on materials, regional focus to develop wearable pieces non-commercially. We are a team of two and can make good use of any 6-shaft loom lying around.
Credits
Exhibited during the exhibition "Fossiles Impossibles"
from the 3rd to the 12th of May 2024
@ Theatre de la Ville in Paris, France
Funded by Sorbonne University
Curated by Justine Jean (DRSCS)
(Directorate of Science, Culture, and Society Relations)
Kaspar Ravel - Artist
Camille Ferrer - Textile Designer
Daniel Santos-Sales - Archaeology and Programming Intern
The code was later curated by Zainab Aliyu of the Processing Foundation
inside the p5.js 2024 Community Sketches collection
It now lives on the front page of the p5.js website